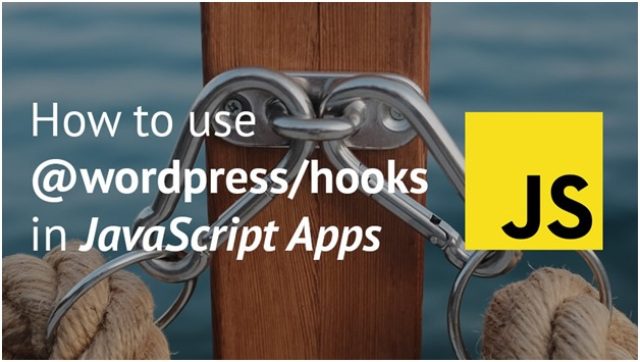
If you want to make your plugins or themes extensible, the best way to do it is to use WordPress packages API (hooks). This is when using PHP. What about when you are using JavaScript? To make your JavaScript project extensible in a similar way, this tutorial will show you how to use those hooks in a React App.
What are @WordPress/hooks?
Let us first define what JavaScript hooks are. When running a JavaScript app in WordPress and you has activated the Gutenberg Editor, you can still use it by calling it from wp.hooks, without installing anything. Another way to go about it is to include that in your JavaScript app by installing it this way:
Npm install @WordPress/hooks –save
How to create your Hook environment
A hook environment is created by building a global object that will be accessible throughout your entire app. Another way is to use the hook system as an internal object which you’ll pass to components.
Import {createHooks} from ‘@WordPress/hooks’;
To start your Hook environment, you can use the create Hooks function this way:
Varmy Global Hooks = create Hooks ();
It is that simple and when you are done, you can start creating actions and filters to make your app extensible.
You can use functions like add action, do action, apply filters, add filter, when you’re used to the WordPress Plugin API. You will, however, notice a small difference when implementing this hook system.
This difference is brought by the namespace which you also add when adding or removing actions and filters. This is applicable for cases where you have the same function names under different components. You can easily distinguish which component or app it belongs to, using namespaces to avoid removing the wrong function by accident.
The available actions functions include:
- addAction( ‘hookName’, ‘namespace’, ‘functionName’, callback, priority )
- removeAction( ‘hookName’, ‘namespace’, ‘functionName’ )
- removeAllActions( ‘hookName’ )
- doAction( ‘hookName’, arg1, arg2, … )
- doingAction( ‘hookName’ )
- didAction( ‘hookName’ )
- hasAction( ‘hookName’ )
Available filter functions are:
- addFilter( ‘hookName’, ‘namespace’, ‘functionName’, callback, priority )
- removeFilter( ‘hookName’, ‘namespace’, ‘functionName’ )
- removeAllFilters( ‘hookName’ )
- applyFilters( ‘hookName’, content, arg1, arg2, … )
- doingFilter( ‘hookName’ )
- didFilter( ‘hookName’ )
- hasFilter( ‘hookName’ )
The string function Name is used to remove the registered callback. However, you can still apply the filter function if it’s not there, but it’s going to be hard removing them later. Another way to view all registered actions and filters through console.log (myGlobalHooks). Here you will also find actions and filters keys. All your actions and filters will be applied here.
Creating the Extensible React App
To kick start a simple React App, lets head straight to “Create React App”. To find out how to use it, you can check the repository information on GitHub.
The first thing you need to do is open your terminal and place it in a folder used on your website or desktop. You then have to create a folder for the app. Remove the my-app name and rename it with a name that suitable for you. The render method in App.js now contains all the JSX that will be rendered. Make that output (JSX) extensible with @WordPress/hooks, so you can combine what you want there.
Ensure that you are inside the newly created folder when installing @WordPress/hooks package:
Npm install @WordPress/hooks>>save
Creating your Hook Environment
You now need to create a global object that will contain your hook environment.
Import React, {Component} from ‘react’;
Import logo from ‘. /logo.svg’;
Import {create Hooks} from ‘@WordPress/hooks’;
Import ‘. /App.css’;
letglobalHooks = createHooks();
Class App extends Component {
// …
}
After that, you will then need to implement some actions and filters. This is done by changing the render method. It will have a variable output that is an array. This is then used with apply Filters to have a few positions where we can add JSX to your output array.
It is clear that you are now using does Action to create a hook where various calls are done before the output even starts. You can later use three apply Filters calls and render your output.
Rendering the Header output
You are now using the whole JSX that you were given in the app creation.
Class App extends Component {
Constructor ( props ) {
// …
globalHooks.addFilter( ‘header_output’, ‘myApp’, this.renderHeader );
};
/**
* Rendering the Header
*
* @param {array} content
*/
renderHeader( header ) {
// Applying filters to change the image. Default is the logo.svg.
letlogoImage = globalHooks.applyFilters( ‘header_logo’, logo );
letshouldLearnReact = globalHooks.applyFilters( ‘should_learn’, true );
letheader_html = <header key=”header” className=”App-header”>
<imgsrc={logoImage} className=”App-logo” alt=”logo” />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
{ shouldLearnReact&&<a
className=”App-link”
href=”https://reactjs.org”
target=”_blank”
rel=”noopenernoreferrer”
>
Learn React
</a>}
</header>;
header.push(header_html );
return header;
}
}
The constructor method allows you to add the filter to the hook header output, with the namespace my App and with the callback to your method render Header. You will receive an array as a parameter to method since the output is an array.
You can then apply different filters, including:
Header logo – you can change the logo
Should learn – This filter is returning a Boolean. Your header will have a link to ReactJS website. By default, it returns true. /li>
Once that is passed through your Hook environment, you will then build a JSX output and push into your array and returning the array. While being inside the app folder, you can type now inside the terminal npm start.
Conclusion
If you are using WordPress then the @WordPress/hooks package is a powerful tool if you are using filters and actions within your plugins/themes or others. This package is the perfect option to help you build extensible JavaScript applications.
About Author:-
Naman Modi is a Professional Blogger, SEO Expert & Guest blogger at NamanModi.com, He is an Award-Winning Freelancer & Web Entrepreneur helping new entrepreneur’s launches their first successful online business.